Message Box
Message box is used to display the information as a pop up.
For example, we want to show the Hello in the message.
Sub Message_Box()
MsgBox ("Hello!")
End Sub
When we run this macro, it will show the Hello message.
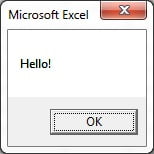
We also can show the button and title in the message like below-
Sub Message_Box()
MsgBox "Hello!", vbInformation, "My Title"
End Sub
When we run this macro, it will show the Hello message along with the information sign and title.
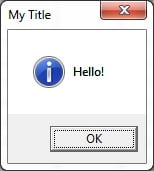
User Confirmation Message Box
We can take the confirmation from the use in Yes and No by using the message box.
Sub Message_Box_confirmation()
Dim s As Integer
s = MsgBox("Do you like VBA???", vbQuestion + vbYesNo)
MsgBox s
End Sub
When we run this code, it will ask question as given in below image-
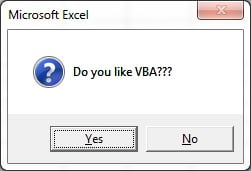
If we click on Yes button, then it will show-
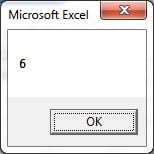
If we click on No button, then it will show-
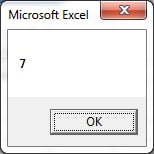
The Buttons parameter can take any of the following values:
- 0 vbOKOnly – Displays OK button only.
- 1 vbOKCancel – Displays OK and Cancel buttons.
- 2 vbAbortRetryIgnore – Displays Abort, Retry, and Ignore buttons.
- 3 vbYesNoCancel – Displays Yes, No, and Cancel buttons.
- 4 vbYesNo – Displays Yes and No buttons.
- 5 vbRetryCancel – Displays Retry and Cancel buttons.
- 16 vbCritical – Displays Critical Message icon.
- 32 vbQuestion – Displays Warning Query icon.
- 48 vbExclamation – Displays Warning Message icon.
- 64 vbInformation – Displays Information Message icon.
The MsgBox function can return one of the following values which can be used to identify the button, user has clicked in the message box.
- 1 – vbOK – OK was clicked
- 2 – vbCancel – Cancel was clicked
- 3 – vbAbort – Abort was clicked
- 4 – vbRetry – Retry was clicked
- 5 – vbIgnore – Ignore was clicked
- 6 – vbYes – Yes was clicked
- 7 – vbNo – No was clicked
Input Box
Input box is used to take the information from user. You can enter your input as number, any text or date.
In the below example we will take the Name as input and will show it as output by using message box.
Sub Input_Box()
Dim s As String
s = InputBox("What is your name?")
MsgBox s
End Sub
Below given pop will be displayed when you will run this code
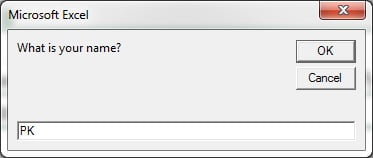
Enter your name and click on OK. Since we have used “msgbox s” so it will display the name which you enter in the message.
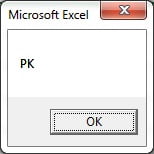
Click here to download the practice file
Watch the step-by-step video tutorial: